注意
前往末尾下載完整範例程式碼。
文字屬性和排版#
使用 Matplotlib 控制文字的屬性和排版。
matplotlib.text.Text
實例具有多種屬性,可以使用關鍵字引數透過 set_title
、set_xlabel
、text
等方法進行配置。
屬性 |
數值類型 |
---|---|
alpha |
|
backgroundcolor |
任何 matplotlib 顏色 |
bbox |
|
clip_box |
matplotlib.transform.Bbox 實例 |
clip_on |
bool |
clip_path |
|
color |
任何 matplotlib 顏色 |
family |
[ |
fontproperties |
|
horizontalalignment 或 ha |
[ |
label |
任何字串 |
linespacing |
|
multialignment |
[ |
name 或 fontname |
字串,例如,[ |
picker |
[None|float|bool|callable] |
position |
(x, y) |
rotation |
[ 以度為單位的角度 | |
size 或 fontsize |
[ 以點為單位的尺寸 | 相對尺寸,例如, |
style 或 fontstyle |
[ |
text |
字串或任何可使用 '%s' 轉換列印的內容 |
transform |
|
variant |
[ |
verticalalignment 或 va |
[ |
visible |
bool |
weight 或 fontweight |
[ |
x |
|
y |
|
zorder |
任何數字 |
您可以使用對齊引數 horizontalalignment
、verticalalignment
和 multialignment
排版文字。horizontalalignment
控制文字的 x 位置引數是否表示文字邊界框的左側、中心或右側。verticalalignment
控制文字的 y 位置引數是否表示文字邊界框的底部、中心或頂部。multialignment
僅適用於以換行符號分隔的字串,控制不同行的文字是否靠左對齊、置中對齊或靠右對齊。以下是一個範例,使用 text()
命令顯示各種對齊方式的可能性。程式碼中通篇使用 transform=ax.transAxes
表示座標是相對於軸邊界框給定的,其中 (0, 0) 是軸的左下角,(1, 1) 是右上角。
import matplotlib.pyplot as plt
import matplotlib.patches as patches
# build a rectangle in axes coords
left, width = .25, .5
bottom, height = .25, .5
right = left + width
top = bottom + height
fig = plt.figure()
ax = fig.add_axes([0, 0, 1, 1])
# axes coordinates: (0, 0) is bottom left and (1, 1) is upper right
p = patches.Rectangle(
(left, bottom), width, height,
fill=False, transform=ax.transAxes, clip_on=False
)
ax.add_patch(p)
ax.text(left, bottom, 'left top',
horizontalalignment='left',
verticalalignment='top',
transform=ax.transAxes)
ax.text(left, bottom, 'left bottom',
horizontalalignment='left',
verticalalignment='bottom',
transform=ax.transAxes)
ax.text(right, top, 'right bottom',
horizontalalignment='right',
verticalalignment='bottom',
transform=ax.transAxes)
ax.text(right, top, 'right top',
horizontalalignment='right',
verticalalignment='top',
transform=ax.transAxes)
ax.text(right, bottom, 'center top',
horizontalalignment='center',
verticalalignment='top',
transform=ax.transAxes)
ax.text(left, 0.5*(bottom+top), 'right center',
horizontalalignment='right',
verticalalignment='center',
rotation='vertical',
transform=ax.transAxes)
ax.text(left, 0.5*(bottom+top), 'left center',
horizontalalignment='left',
verticalalignment='center',
rotation='vertical',
transform=ax.transAxes)
ax.text(0.5*(left+right), 0.5*(bottom+top), 'middle',
horizontalalignment='center',
verticalalignment='center',
fontsize=20, color='red',
transform=ax.transAxes)
ax.text(right, 0.5*(bottom+top), 'centered',
horizontalalignment='center',
verticalalignment='center',
rotation='vertical',
transform=ax.transAxes)
ax.text(left, top, 'rotated\nwith newlines',
horizontalalignment='center',
verticalalignment='center',
rotation=45,
transform=ax.transAxes)
ax.set_axis_off()
plt.show()
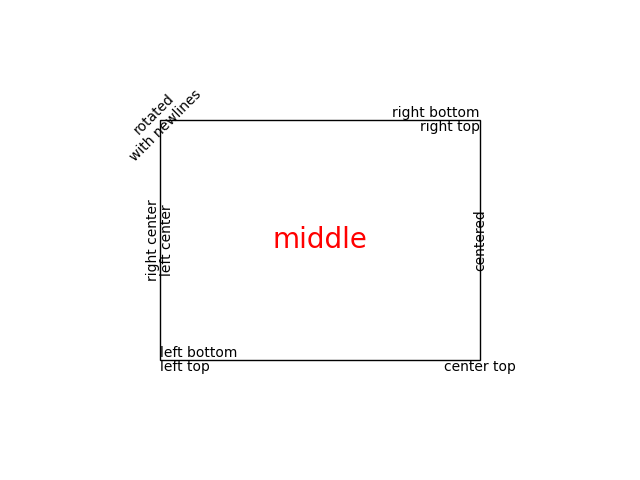
預設字型#
基本預設字型由一組 rcParams 控制。若要設定數學表達式的字型,請使用以 mathtext
開頭的 rcParams(請參閱 mathtext)。
rcParam |
用途 |
---|---|
|
字型系列清單(安裝在使用者機器上)和/或 |
|
預設樣式,例如 |
|
預設變體,例如 |
|
預設拉伸,例如 |
|
預設權重。字串或整數 |
|
以點為單位的預設字型大小。相對字型大小( |
Matplotlib 可以使用安裝在使用者電腦上的字型系列,例如 Helvetica、Times 等。也可以使用泛型系列別名指定字型系列,例如({'cursive', 'fantasy', 'monospace', 'sans', 'sans serif', 'sans-serif', 'serif'}
)。
注意
若要存取可用字型的完整清單
matplotlib.font_manager.get_font_names()
泛型系列別名與實際字型系列之間的映射(在 預設 rcParams 中提到)由以下 rcParams 控制
基於 CSS 的泛型系列別名 |
具有映射的 rcParam |
---|---|
|
|
|
|
|
|
|
|
|
|
如果任何泛型系列名稱出現在 'font.family'
中,我們會將該項目取代為對應 rcParam 映射中的所有項目。例如
matplotlib.rcParams['font.family'] = ['Family1', 'serif', 'Family2']
matplotlib.rcParams['font.serif'] = ['SerifFamily1', 'SerifFamily2']
# This is effectively translated to:
matplotlib.rcParams['font.family'] = ['Family1', 'SerifFamily1', 'SerifFamily2', 'Family2']
具有非拉丁字形的文字#
自 v2.0 版本起,預設字型 DejaVu 包含了許多西方字母的字形,但不包含其他文字,例如中文、韓文或日文。
若要將預設字型設定為支援您所需碼位的字型,請將字型名稱加在 'font.family'
的前面(推薦做法),或加在想要的別名字型列表前面。
# first method
matplotlib.rcParams['font.family'] = ['Source Han Sans TW', 'sans-serif']
# second method
matplotlib.rcParams['font.family'] = ['sans-serif']
matplotlib.rcParams['sans-serif'] = ['Source Han Sans TW', ...]
通用字型別名列表包含與 Matplotlib 一起發行的字型(因此 100% 可以找到),或是在大多數系統中很可能存在的字型。
設定自訂字型系列時,一個好的做法是在字型系列列表的最後追加一個通用字型作為最後的備用方案。
您也可以在 .matplotlibrc
檔案中進行設定。
font.family: Source Han Sans TW, Arial, sans-serif
若要控制每個藝術家 (artist) 使用的字型,請使用 文字屬性與佈局 中描述的 name、fontname 或 fontproperties 關鍵字參數。
在 Linux 上,fc-list 是一個有用的工具,可以用來尋找字型名稱;例如:
$ fc-list :lang=zh family
Noto to Sans Mono CJK TC,Noto Sans Mono CJK TC Bold
Noto Sans CJK TC,Noto Sans CJK TC Medium
Noto Sans CJK TC,Noto Sans CJK TC DemiLight
Noto Sans CJK KR,Noto Sans CJK KR Black
Noto Sans CJK TC,Noto Sans CJK TC Black
Noto Sans Mono CJK TC,Noto Sans Mono CJK TC Regular
Noto Sans CJK SC,Noto Sans CJK SC Light
會列出所有支援中文的字型。